ssapy.utils
This module provides functions for coordinate transformations, vector operations, celestial mechanics, and mathematical utilities.
Functions
|
Convert altitude angle to zenith angle. |
|
Calculates the angle (in radians) between two vectors. |
|
Convert Cartesian coordinates to spherical coordinates in degrees. |
|
Convert Cartesian coordinates to cylindrical coordinates. |
|
Convert ra/dec of stars from catalog positions (J2000/ICRS) to apparent positions, correcting for proper motion, parallax, annual and diurnal aberration. |
|
Checks if the trajectory of a particle intersects with the Moon. |
|
Down-select emcee walkers to those with the largest posterior mean. |
|
Re-open the decorated class, adding any new definitions into the original. For example: .. code-block:: python class Foo: pass @continueClass class Foo: def run(self): return None is equivalent to: .. code-block:: python class Foo: def run(self): return None .. warning:: Python's built-in super function does not behave properly in classes decorated with continueClass. Base class methods must be invoked directly using their explicit types instead. |
|
Converts a Decimal Degree (DD) value to Degree-Minute-Second (DMS) format. |
|
Converts Decimal Degrees (DD) to Hour-Minute-Second (HMS) format. |
|
Normalize angles in degrees to the range [0, 360]. |
|
Normalize an array of angles in degrees to the range [0, 360]. |
|
Normalize angles to the range [-90, 90]. |
|
Normalize an array of angles to the range [0, 90]. |
|
Converts coordinates from Degree-Minute-Second (DMS) format to Decimal Degrees (DD). |
|
Convert coordinates from degrees, minutes, and seconds (DMS) format to decimal degrees. |
|
Convert coordinates from degrees, minutes, and seconds (DMS) format to radians. |
|
Convert ecliptic coordinates (longitude, latitude) to equatorial right ascension (RA) and declination (DEC). |
|
Convert ecliptic Cartesian coordinates (X, Y, Z) to equatorial right ascension (RA) and declination (DEC). |
|
Convert ecliptic rectangular coordinates (X, Y, Z) to equatorial rectangular coordinates. |
|
Compute the norm of an array using Einstein summation notation with customizable indices. |
|
Convert equatorial coordinates (RA, DEC) to ecliptic longitude and latitude. |
|
Convert equatorial coordinates (declination and either right ascension or hour angle) to horizontal coordinates (azimuth and altitude) for a given observer's latitude. |
|
Convert equatorial rectangular coordinates (X, Y, Z) to ecliptic rectangular coordinates. |
|
Attempt to find all zeros of a function between given bounds. |
Find triplets bracketing extrema from an ordered set of function evaluations. |
|
|
Find a file in the current directory or the ssapy datadir. |
|
Finds the indices of the nearest values in array A for each value in array B. |
|
Find the smallest bounding cube for a set of 3D coordinates, with optional padding. |
|
Convert position vectors from the GCRF (Geocentric Celestial Reference Frame) to the ECEF (Earth-Centered, Earth-Fixed) frame. |
|
Converts a position vector in the GCRF (Geocentric Celestial Reference Frame) to the ITRF (International Terrestrial Reference Frame) in Cartesian coordinates. |
|
Converts position vectors from the GCRF (Geocentric Celestial Reference Frame) to the ITRF (International Terrestrial Reference Frame) using Astropy. |
|
Converts a position vector in the GCRF (Geocentric Celestial Reference Frame) to latitude, longitude, and height coordinates on Earth. |
|
Transform position and velocity vectors from the GCRF (Geocentric Celestial Reference Frame) to a lunar-centric frame. |
|
Transform position and velocity vectors from the GCRF (Geocentric Celestial Reference Frame) to a Moon-fixed (lunar-centric) frame. |
|
|
|
Transforms a position vector in the GCRF (Geocentric Celestial Reference Frame) to a simplified geostationary-like coordinate system. |
|
Return the rotation matrix that converts GCRS cartesian coordinates to TEME cartesian coordinates. |
|
Calculate the angle between two vectors where b is the vertex of the angle. |
|
Get the covariance matrix of kernel_mat. |
|
Computes normalized weights from log-weights. |
|
Calculate a list of times spaced equally apart over a specified duration. |
|
Converts Hour-Minute-Second (HMS) format to Decimal Degrees (DD). |
|
Convert horizontal coordinates (azimuth and altitude) to equatorial coordinates (hour angle and declination). |
|
Interpolate IERS values |
|
Transform inertial coordinates to rotated coordinates relative to the Earth. |
Interpolates points between the given points. |
|
|
Return True if an attribute is safe to monkeypatch-transfer to another class. |
|
Convert lb-like coordinates & proper motions to orthographic tangent plane. |
|
Convert lb-like coordinates to theta-phi like coordinates. |
|
Convert lb-like coordinates to unit vectors. |
|
Calculate the great-circle distance between two points on Earth's surface using the Haversine formula. |
|
Compute GCRF position of the moon. |
|
Find a root of a univariate function with known gradient using Newton-Raphson iterations. |
|
Compute the Euclidean norm of an array over the last axis while preserving leading axes. |
|
Compute the squared norm of an array over the last axis while preserving leading axes. |
|
Normalize an array along the last axis to have unit length. |
|
Convert NTW coordinates to cartesian coordinates, using r, v to define NTW system. |
|
Return number of times angle ang has wrapped around. |
Returns two vectors that are perpendicular to v and each other. |
|
|
Generate points on a circle in 3D space. |
|
Calculate the proper motion in right ascension (RA) and declination (DEC) for celestial objects. |
|
Calculate the Right Ascension (RA) and Declination (Dec) of an object relative to Earth's position. |
|
Normalize angles in radians to the range [0, 2π]. |
|
Perform particle regularization. |
|
Resample particles to achieve more uniform weights. |
|
Convert right ascension to hour angle. |
|
Rotate a set of 3D points about a 3D axis by an angle theta in radians. |
|
Rotates a unit vector by specified angles and optionally plots the rotation path. |
|
Find the rotation matrix that aligns vec1 to vec2 :param vec1: A 3d "source" vector :param vec2: A 3d "destination" vector :return mat: A transform matrix (3x3) which when applied to vec1, aligns it with vec2. |
|
Convert coordinates to NTW coordinates, using r, v to define NTW system. |
|
Sample points around x according to covariance matrix. |
|
Compute f(sigma points) for sigma points of C around x. |
|
Simulate longitude, latitude, and radius in a geocentric frame with the Sun at (0, 0). |
|
Select MCMC samples with probabilities above some relative threshold |
|
Compute GCRF position of the sun. |
|
Calculate the Right Ascension (RA) and Declination (Dec) of the Sun at a given time. |
|
Convert orthographic tangent plane coordinates to lb coordinates. |
|
Return the rotation matrix that converts TEME cartesian coordinates to GCRS cartesian coordinates. |
|
Convert theta-phi-like coordinates to lb-like coordinates. |
|
Convert theta-phi-like coordinates to unit vectors. |
|
Robustly compute angle between unit vectors r1 and r2. |
|
Convert unit vectors to lb-like coordinates. |
|
Convert unit vectors to theta-phi-like coordinates. |
|
Returns the unit vector of the vector. |
|
Compute mean and covariance matrix using unscented transform given a transformation f, a point x, and a covariance C. |
|
Calculate the velocity from position and time data. |
|
Convert rectangular coordinates (X, Y, Z) to ecliptic longitude and latitude. |
|
Convert rectangular coordinates (X, Y, Z) to equatorial right ascension (RA) and declination (DEC). |
|
Convert x, y, z vectors to lb-like coordinates. |
|
Convert x, y, z vectors to theta-phi-like coordinates. |
|
Convert zenith angle to altitude angle. |
Classes
|
Simplified Least Recently Used Cache. |
|
Represent and manipulate times and dates for astronomy. |
|
meant to be used for lazy evaluation of an object attribute. |
Class Inheritance Diagram
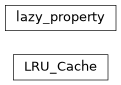